Getting Started with Ruby: Guide for Beginners
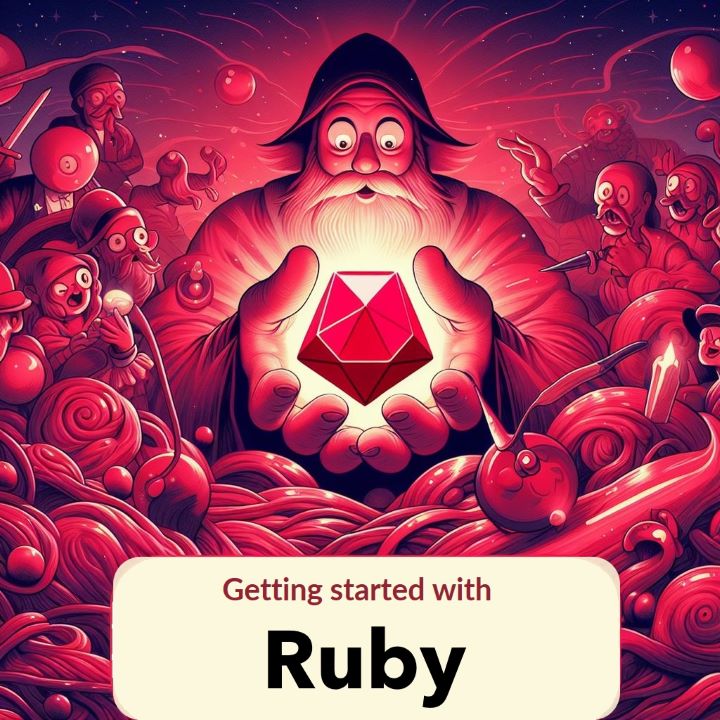
Ruby is a dynamic, reflective, object-oriented programming language known for its simplicity and readability. Whether you’re new to programming or looking to add a versatile language to your skill set, Ruby is an excellent choice. In this guide, we’ll walk you through the basics of Ruby programming, from setting up your environment to writing your first program.
Installing Ruby
Before diving into Ruby programming, you need to install Ruby on your machine. Follow these steps based on your operating system:
For macOS and Linux:
- Open your terminal.
- Run the following command to check if Ruby is already installed:
ruby -v
- If Ruby is not installed, you can install it using a version manager like RVM or rbenv. Choose one and follow the installation instructions on their respective websites.
For Windows:
- Download the RubyInstaller from the official Ruby website or directly go to https://rubyinstaller.org/ .
- Run the installer and follow the on-screen instructions.
- Make sure to check the option to add Ruby to your system’s PATH during installation.
Once the installation is complete, open a new terminal window to verify that Ruby has been installed successfully.
Your First Ruby Program
Let’s start by writing a simple “Hello, World!” program to ensure everything is set up correctly. Open your text editor and create a new file named hello.rb
. Enter the following code:
puts "Hello, World!"
Save the file and go back to your terminal. Navigate to the directory where you saved hello.rb
and run the program using the following command:
ruby hello.rb
You should see the output “Hello, World!” printed in the terminal. Congratulations! You’ve just written and executed your first Ruby program.
Understanding Ruby Basics
Variables and Data Types
In Ruby, variables are dynamically typed, meaning you don’t have to explicitly declare their data type. Here’s an example:
# Variable assignment
name = "John"
age = 25
# Output
puts "Name: #{name}, Age: #{age}"
In this example, name
is assigned a string value, and age
is assigned an integer value. The puts
statement is used to print the values to the console.
Control Flow
Ruby provides standard control flow structures like if
, else
, and elsif
for decision-making. Here’s an example:
# Control flow example
score = 85
if score >= 90
puts "A"
elsif score >= 80
puts "B"
else
puts "C"
end
In this example, the program evaluates the value of score
and prints the corresponding grade.
Loops
Ruby supports various loop constructs, with the while
loop being one of the most common. Here’s a simple example:
# While loop example
count = 0
while count < 5
puts "Count: #{count}"
count += 1
end
This loop will print the value of count
until it reaches 5.
Arrays and Hashes
Ruby provides two main data structures for storing collections of data: arrays and hashes.
Arrays
# Array example
fruits = ["apple", "orange", "banana"]
# Accessing elements
puts fruits[0] # Output: apple
# Iterating through array
fruits.each do |fruit|
puts fruit
end
Hashes
# Hash example
person = { "name" => "Alice", "age" => 30, "city" => "Wonderland" }
# Accessing values
puts person["name"] # Output: Alice
# Iterating through hash
person.each do |key, value|
puts "#{key}: #{value}"
end
Methods
In Ruby, you can define methods to encapsulate reusable pieces of code. Here’s an example:
# Method example
def greet(name)
puts "Hello, #{name}!"
end
# Call the method
greet("Bob") # Output: Hello, Bob!
Next Steps
This guide covers the basics of setting up Ruby, writing a simple program, and understanding fundamental language features. As you continue your Ruby journey, explore more advanced topics such as classes, modules, and Ruby on Rails for web development.
The official Ruby documentation and RubyGuides are excellent resources for further learning. Happy coding!
Comments